Store variables in cookies in PHP
In a cookie you can store very little data (up to 4093 characters per cookie), and it is only possible to store up to 20 cookies per domain (this is important to keep in mind if in different sessions of our site we use cookies), and a browser can only store up to 300 cookies in total.
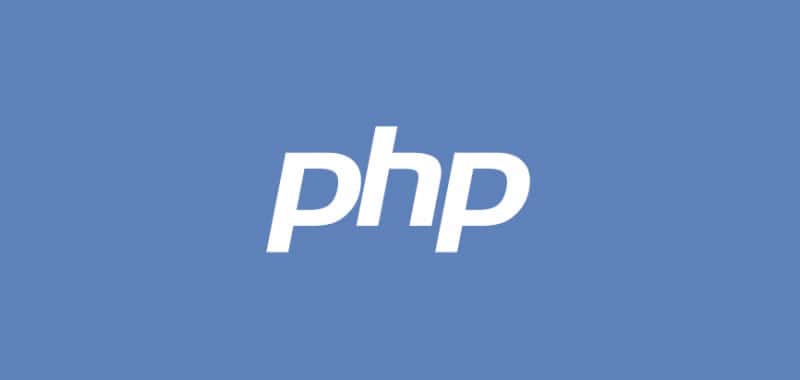
In a cookie you can store very little data (up to 4093 characters per cookie), and it is only possible to store up to 20 cookies per domain (this is important to keep in mind if in different sessions of our site we use cookies) , and a browser can only store up to 300 cookies in total, shared among all the sites visited by that user (otherwise, the browser will begin to delete the oldest cookies, which is the reason why some sites use cookies to remember our data, ask us again and again to identify ourselves, periodically: it is because your cookie was deleted, displaced by other newer cookies from other sites that we visited
Due to this "scarcity" of resources of the cookies, it is very common to store only one code in the cookie, so that it identifies that user in that browser on that computer, and the rest of the associated data is stored in the server (in the hosting, usually in database).
An old myth (more than ten years, an eternity in the history of the web!) Generates all kinds of rumors about cookies: it is said that they reveal our private data, that spy our computer, and many other fantasies, caused for the ignorance of its true limits.
The only real capacity of a cookie is to store a few data in variables, which we can then use when, from the same machine and using the same browser, that user again enters our web page.
This almost trivial detail deserves to be emphasized: it is not the user itself, and it is not even the user's computer that is identified, but only the browser that was used; if from the same computer the same website or the brother or co-worker of our user entered using the same browser, we would confuse it with our user. And, on the contrary, if the same user enters using another browser, or from another computer, we will not recognize it ... and something else: you can only read the cookie from the domain that created it, so from "espionage", little and nothing. Except in case too sophisticated, which are only possible for large online advertising companies, which host their banners in the same domain but publish them in different sites and, in this way, detect the code of their cookie across several sites, and they can take statistics of the profile of visits of a user: if I visit such newspaper, and then such another site of auctions, etc. But that only creates profiles to show advertisements related to the user, and not individualizable data with name and surname (except for exceptions that, like witches, do not exist, but "there are, there are ..." although they require that some of the sites the chain share their databases, something that enters the terrain of the legal rather than the programming).
There are some considerations to keep in mind before moving on to the code.
The cookies are generated through an order that must reach the user's browser before it processes the HTML code that will make us see a page on our screen. To achieve this, the order for the creation of the cookie must travel in the header or header of the HTTP address that brings us the HTML file, from the server to the browser; so the execution of the function that creates a cookie file must be before any HTML code or any echo of PHP that already on the page (as with the sessions, as we will see soon), so that it arrives within the headers of the HTTP request.
In the following example we will see what would be the location of the code that creates a cookie:
<?php
setcookie("name" ,"John");
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"
"http://www.w3.org/TR/xhtmll-strict.dtd">
<html ...etc ...
</html>
Let's analyze the preceding code. The setcookie function, included in the PHP language, receives as the first "argument" (first thing in its parentheses) the name of the variable that will be stored in the cookie and, as a second argument (separated by one as in the previous argument), the data that you want to store within that variable, which the browser will store in a text file on the user's computer:
Setcookie("nombre", "John");
The setcookie function allows you to specify several other arguments within their parentheses, but they are all optional:
Setcookie(nombre , valor, duracion, ruta, dominio, seguridad)
Some of them we will see some paragraphs later. But with these two data (the name of the variable and its value), separated by commas and wrapped in quotes, it is enough to create a cookie and save a piece of information.
This example, which defines a variable called "name" with the value "Juancito", has actually created a cookie (try running it on our local server, or on our hosting). As you can not see anything special on the screen, we have the doubt: we do not know if you have managed to create this cookie, or not.
Fortunately, it is easy to check if it is believed, or not: we can see with our own browser all the cookies that browser has stored, and what is the value that keeps inside each of them.
In each browser, the way to access cookies is different; For example, in Firefox, we access the menu Tools → Options → Privacy → Delete cookies individually, which shows us the list of cookies that exists in that browser, as well as its content, its expiration date and other complementary information.
In this case, it would look like this:
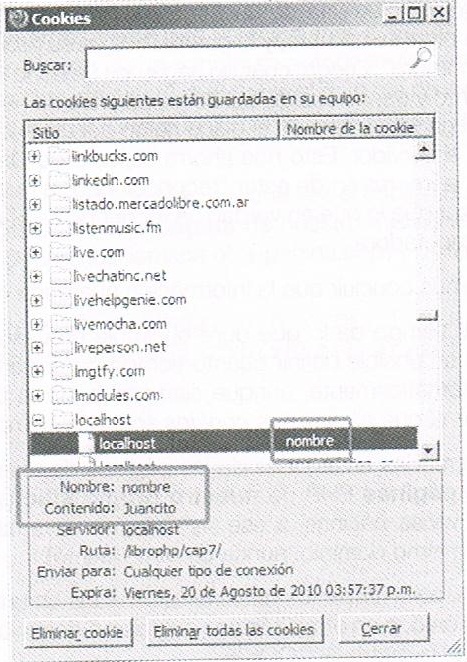
In this way, we verify if the cookie was created and with what value. This is very useful while we program and we need to detect if our code worked or if there were errors. But of course, the most usual way to work with the data stored in cookies is to read them and use them directly from the PHP code that we program on our website and we will learn below.
Read cookie variables
We will try to understand how is the reading circuit of an inside that was in a cookie. As we said, the cookie is automatically returned by the browser to the server (hosting) every time the browser detects that a request is being made to a URL of a domain that already has a cookie stored in that same browser, that is, in When we click on a link, we send a form or write a URL and press enter, if any of these three actions points to a site that previously saved a cookie, at that same moment, our browser sends the variables contained in the cookie to the server corresponding to that site (they are going to use it or not on that server, which is not a matter that interests the browser, he only sends them without asking), without needing to do anything special, everything happens invisibly and automatically .
Those variables stored in cookies that are sent to the server, are stored by the Web server is an array, as happened with the data sent by get links or by post form, only this time the array is called $_COOKIE.
Imagine an example: in one of our pages, we ask the user to fill in his name with a form, and we save it on his own machine, in a cookie variable called "name". then when we do an echo $ _COOKIE ["name"] at any other time and from any of the pages of our site, this will be possible thanks to the browser already read on the user's hard disk this variable and automatically forward to the server.
This saves us storage space in our database, and causes the impression of being "recognizing" our users as soon as they enter our site ... although what is really recognized is the file that we have left stored on that computer.
Therefore, we can conclude that the information stored in cookies:
- • It lasts longer than the simple process of a PHP page lasts (we will see that it is possible to define how long it will last before the browser automatically deletes it, although there is always the possibility that it is the user who deletes their cookies before of the planned time)
- • Cookies created in some pages of our site, can be read from other PHP pages of our own site (which allows to identify, over several pages, that visitor, always talking about PHP pages within the same domain, never of another different).
Returning to the code, to use the data stored in a cookie on our pages (in the same one that created it, or in other pages of the same domain), we simply have to show a cell of the $ _COOKIE matrix.
Let's try the creation of this page, and let's call it initial.php:
<?php
setcookie ("my variable", "data, data and more data", time()+60);
//Remember that this is essential to be at the beginning of the file
?>
<!DOCTYPE html PUBLIC "-//W3C// DTC XHTML 1.0 strict// EN2
" http: // www.w3. Org/ TR/ xhtm11 /DTD/ xhtm11-strict.dtd">
<html>
<head>
… etc…
</head>
<body>
<h1> the cookie has already been created!<h1>
</body>
</html>
Through the code, we define in the user's machine a variable called "myvariable", in which we store a text that says "data, data and more data".
The third argument of the setcookie function specifies the duration or the expiration time of the cookie; that is, when the user's browser should remove the text file from its hard drive.
In this case, the current moment has been passed as the third argument (the time () function, which we will see later in this article) plus 60 seconds, this means that after a minute that cookie will be destroyed. If we try to read after the 60 seconds, the data will not be available, it will no longer exist (if we do not specify any erase, the cookie will be automatically deleted as soon as the user closes his browser).
If we want to try it after executing once the code that we created to the cookie, we enter with our browser on another page of our server that contains the reading of that variable, we could call it another-page.php, and it would contain something like this:
<¡DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 strict //EN"
"http: www.w3 .org/ TR/xhtm11//DTD/XHTML11-strict.dtd">
<html>
<head>
…etc…
</head>
<body>
<h1>
<?php
if(isset($_COOKIE["myvariable"] ) ) {
echo "The cookie contains: ";
echo $_COOKIE[" myvariable"];
} else {
Echo " It seems that it did not go through the homepage. PHP, Go back to it that way of creating the cookie " ;
}
?>
</h1>
</body>
</html>
If instead of doing in two different pages this use of cookie data, we would like to do it in a single page, the code would be similar to the following (we can call this page in-a-single.php):
<?php
if(isset($_COOKIE["mivariable"])) {
//If it is not present, we create it:
setcookie("myvariable", "data, data and more data", time()+60);
}
?>
<!DOCTYPE html PUBLIC "- //W3C//DTD XHTML 1.0 Strict // EN"
"http: //www.w3.org/ xhtm11 / DTD/ xhtm11-strict.dtd">
<html>
<head>
…etc…
</head>
<body>
<h1>
<?php
if (isset($_COOKIE[ "mivariable"] ) ) {
//if available, we use it:
echo "The cookie contains: ";
echo $_COOKIE["myvariable"];
} else {
echo "As it is the first time you enter this page, the order to create the cookie has just been sent to the browser, along with this code and text that you are reading, if you request this page again by pressing F5, the browser will send the server the cookie and may be read and used.";
}
?>
</h1>
</boby>
</html>
NOTE: We can not read a cookie at the same time that we order it to be created with the setcookie function, but just from the next request to the server, the value of the cookie will be sent by the browser to the server and there it can be used.
ChatGPT Free
Ask questions on any topic
CITE ARTICLE
For homework, research, thesis, books, magazines, blogs or academic articles
APA Format Reference:
Delgado, Hugo. (2019).
Store variables in cookies in PHP.
Retrieved Dec 30, 2024, from
https://disenowebakus.net/en/storing-variables-cookies-php