Validations in PHP
In this dialogue in stages that involve the dynamic pages, this waiting on a second page is a data that the user should have sent to the server from a previous page (either with a link that sends variables, or with a form), it may happen that the expected data will never arrive, which would frustrate the attempt to use it.
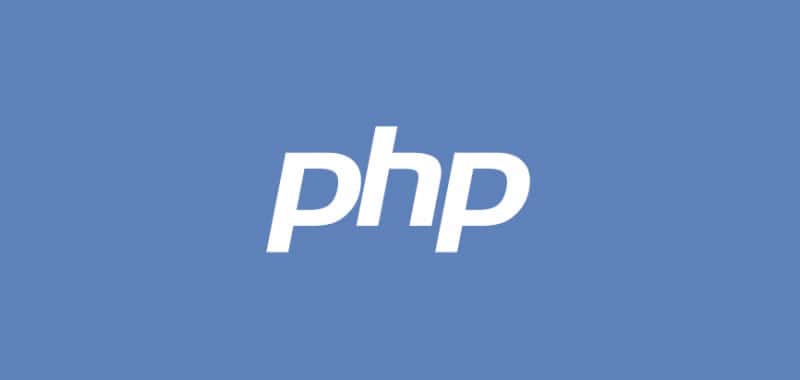
In this "dialogue in stages" that involve the dynamic pages, this "waiting" on a second page is a data that the user should have sent to the server from a previous page (either with a link that sends variables, or with a form), it may happen that the expected data never arrives, which would frustrate the attempt to use it.
Let's go back to analyzing the code of a previous example: suppose we have a page called form.html that contains this:
<form action="sample.php" method="post">
<input type= "text" name="domicile"/>
<input type="submit" value="Send"/>
</form>
And we also have a page called sample.php, which is the page where we will be waiting for the variable "domicile", to use it:
<?php
print("your address is:");
print($_POST["domicile"]);
?>
This scheme is taking for granted an ideal situation, an expected way to navigate these two pages, which will not necessarily be the path that every user will follow. We give of course the following:
1. That the user will first enter form.html,
2. That he will write something in the field called "address",
3. Then press the send button,
4. That you will receive instantaneously later as a response the result of the procedure of the page “sample.php”, that will show you your address.
Posiblemente, ese sea el comportamiento de la mayoría de los usuarios, pero... y que pasara con las exposiciones que no sigan ese camino ideal?
Possibly, that is the behavior of most users, but ... and what happens with exhibitions that do not follow this ideal path? What would happen if a user who already uses these two pages and knows the two URLs (or keep them in their favorites), goes directly to muestra.php, without having gone through the form before? That is, if it directly enters the one, we assumed was going to be the "second step" of the series of steps, skipping the first one.
What you will get will be an error message similar to this:
Your address is:
Notice: Undefined index: domicile in ... etc.
That is, no cell called "domicile" is found within the $ _POST array that will be trying to read, in the destination file, when we order the PHP interpreter to show its value with the print command ($_POST ["domicile"] "]);
Needless to say, the error messages of the PHP interpreter are something that should never appear on our pages: if we have programmed our code well, we could avoid those errors completely.
Avoiding that error is very simple, it is a matter of anticipating the possibility of absence of this expected data, first checking if the data is present. Only if it is present, we will proceed to use it and, conversely, we will show some indication to the user (for example, a link to the form that should have been used).
The problem in this situation is that you are trying to read the $_POST["home"] cell.
And, having not gone through the form before, that cell does not exist directly. And you cannot read something that does not exist.
To anticipate the possibility of absence of an expected data, we will use a conditional, which verifies if the variable or matrix cell that we are about to use is defined; if it is present, in that case we will use it.
To verify if a variable or matrix cell is defined, we will use the following syntax:
<?php
if (isset($_POST["domicile"]) ){
print ("<p> su dirección es : ");
print ($_POST["domicilio"]);
print ("</p>");
} else {
print ("<p>Oops! It seems I do not pass through the <a
href=\"formulario.html\"> form</a>. please, stop by and send us your address.</p>
}
?>
Note that we have foreseen the two possibilities:
• That it is present, that is, that the data "domicile" has already been sent (in which case, we use it). OR
• That it has not been provided, in which case we ask the user to go through the form and use it.
We will always use this validation when we are waiting for the user to provide a data, since it is possible that this does not reach us. For this reason, we must anticipate that possibility so as not to end up with an error message.
If we want to validate the arrival of more than one variable at the same time, we can unite with more the names of those variables (or cells of matrices) within the isset:
<?php
if(isset($_POST["nombre"],$_POST["apellido"],$_POST["edad"])){
// Solo si todos estos datos están presentes, devuelve verdadero y se ejecuta lo que este entre las llaves del if
}
We could also join different isset with logical operators:
<?php
if (isset($_POST["nombre"])and isset($_POST["apellido"])and isset $_POST["edad"])){
// Solo si todos estos datos están presentes, devuelve verdadero y se ejecuta lo que este entre las llaves del if
}
The validation of "if a data is defined", is the most necessary of all, and we will use it whenever we expect to receive a data from the user's side and, therefore, it could happen that it is not provided, that it is not I presented.
If not, it is empty
Another possibility directly (although it seems to be the same as the previous one, it is not) is that the user does pass through the form, but I sent it to the server without having written anything inside the field. That is, the expected data if it will be "defined", will have reached the server, but with a value of "nothing", totally empty.
The difference between "being defined" and "being empty" is similar to the difference between the fact that a letter has arrived in our mailbox and the letter is empty.
• If a variable is not defined, it means that no letter arrived.
• Being defined means that the letter arrived (but it may still be full or empty, until we open it, we will not know).
• Being empty assumes that it arrived (it is defined), but without content.
• Being full is the ideal situation: I arrive (it is defined) and it has content (it is not empty).
Validating this possibility is possible through the comparison operations that we have used in other occasions. Depending on the way we express the condition, we can use different comparison operators. We may want to know if something is empty, and in that case we will write the condition:
<?php
if ($_POST["domicilio"] == "") {
// si es cierto, es que está vacio
}
?>
And, in other cases, if we want to know if something is not empty, we can use both the operator <> and the operator! =, Either:
<?php
if ($_POST["domicilio"] <> "") {
// si es cierto, es que No está vacio
}
?>
Or what is the same:
<?php
if ($_POST["domicilio"] != "") {
// si es cierto, es que No está vacio
}
?>
Note that we have used the navigation operator! so that the condition is true if "is not equal" to empty (and we express the empty value by "nothing" in quotes, literally nothing, not even an empty space between the opening and closing of the quotes).
In this way, we will know if a data has been filled with some value by the user before using it in our code.
Applying the previous example, we could change both validations in a single one (that the data is present, and that is not empty) by means of a logical operator with both conditions:
<?php
if(isset($_POST["domicilio"]) and $_POST["domicile"] !=""){
print("<p> Your address is: ");
print($_POST["domicile"]);
print("</p>");
} else {
print ("<p>Oops! It seems like I did not go through the <a href=\"form.html\">form</a>. please, go ahead and write your address.</p>");
}
?>
If its value is within a range
Other times, we will need to compare values (usually numerical) to know if they are within a range of possible values. For example, if the variable "month" of a form of a calendar is a number greater than zero and less than 13 (the twelve months of the year):
<?php
$month=10; //suppose this would come from a form
if($month > 0 and $month < = 12) {
print ("<p>You chose the month: $month");
} else {
print ("</p> You have chosen a nonexistent month <p/>");
}
?>
It is important that we observe how we have used the logical operator and to define a range by joining two different conditions: that $ month is greater than zero (first condition), and that $ month is less than or equal to 12 (second condition). With these two limits, we have defined a range of allowed values. If both conditions are true, the code wrapped between the keys of the if is executed; otherwise, the wrapped between keys of else is executed.
If instead of a single range, it is a series of range of values, we should use a series of else.
If, instead of a single rank, it were a series of range of values, we should use a series of elseifs:
<?php
$total =145; // suppose this would come from a form
if($total > 0 and $total <= 500){
if($total <= 100){
print ("¡Very cheap! Less than 100 pesos ...");
} elseif ($total<= 200){
print ("Good price, between 101 and 200 pesos.");
} elseif ($total<= 500){
print ("Somewhat expensive, between 201 and 500 pesos.");
}
}else{
print ("The value is outside the allowed range of 1 to 500");
}
?>
Notice how we are "nesting" different levels of conditions: the first condition defines the possible range between 1 and 500, and only in case of being within that range, it goes to the next level of validation (to evaluate exactly in which price range) it is located).
That first condition has an else that communicates a message to the user in case of having entered a total outside the allowed range.
But, once verified that the value of $ total is between 1 and 500, we proceed to a series of validations excluding, connected, of which only one of the ranges will be true: or is less than 100, or is between 101 and 200, or is between 201 and 500. There is no other possibility.
At this point, we are able to make full validations to all the data we expect the user to send to the server.
ChatGPT Free
Ask questions on any topic
CITE ARTICLE
For homework, research, thesis, books, magazines, blogs or academic articles
APA Format Reference:
Delgado, Hugo. (2019).
Validations in PHP.
Retrieved Dec 14, 2024, from
https://disenowebakus.net/en/validations-types-php